Pardot forms are a powerful tool for capturing leads and gathering information from prospects. However, one common issue arises when dealing with the state field: it cannot dynamically display states based on the country selected by the user. In this blog post, we’ll explore a solution to this problem by customizing the state field dropdown to show only relevant states for specific countries.
The Problem
When creating a Pardot form, you might encounter situations where you want to display a state field (e.g., for addresses) but limit the available options based on the user’s chosen country. Unfortunately, out of the box, Pardot does not provide a straightforward way to achieve this dynamic behavior.
The Solution
Demo of the solution is in below form: Please select United states/Canada to see relavent state values
To address this limitation, follow these steps to create a Pardot form that displays the correct state options for each country:
Step 1: Add the State Field
- Log in to Pardot and navigate to the relevant form.
- Click on Form Fields and add a new field for the state. Configure it as follows:
- Prospect Field: State
- Type: Dropdown
- Required: Unchecked
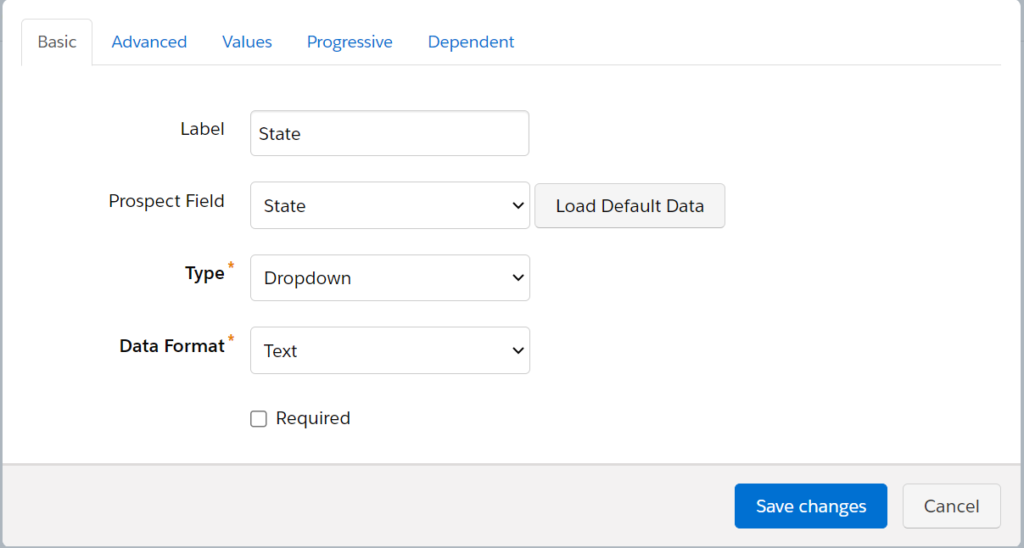
- In the Values section, add all the states you want to display for the countries you’re targeting.
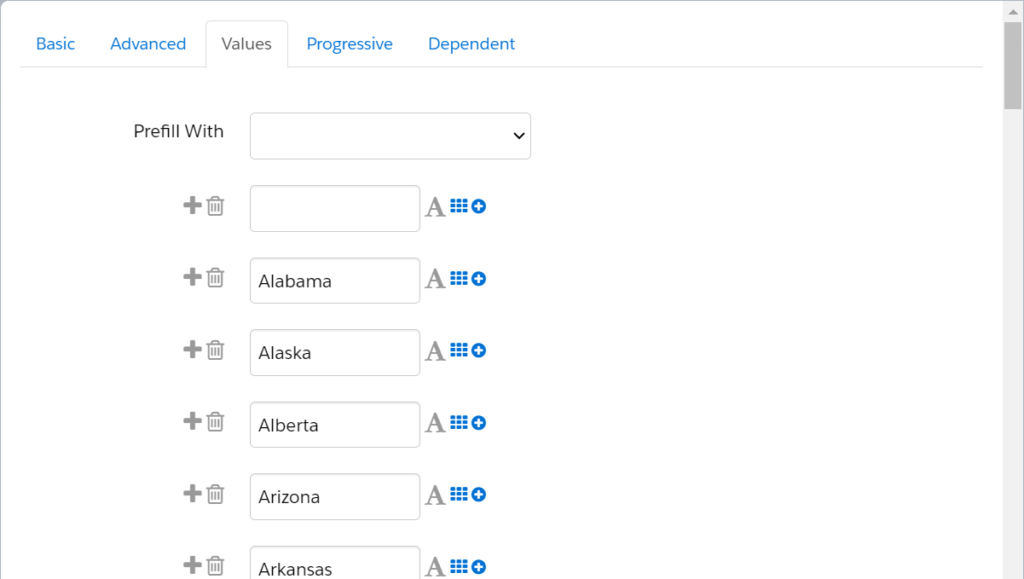
Step 2: Custom Code in Look and Feel
- Go to the Look and Feel section of your Pardot form.
- Under the Form tab, select the Code option.
- Paste the following code snippet:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script>
var countryStateMap = {
"unitedstates": {
"countryName": "US",
"states": [
{
"value": "Alaska",
"displayValue": "Alaska"
},
{
"value": "Alabama",
"displayValue": "Alabama"
},
{
"value": "Arkansas",
"displayValue": "Arkansas"
},
{
"value": "Arizona",
"displayValue": "Arizona"
},
{
"value": "California",
"displayValue": "California"
},
{
"value": "Colorado",
"displayValue": "Colorado"
},
{
"value": "Connecticut",
"displayValue": "Connecticut"
},
{
"value": "District of Columbia",
"displayValue": "District of Columbia"
},
{
"value": "Delaware",
"displayValue": "Delaware"
},
{
"value": "Florida",
"displayValue": "Florida"
},
{
"value": "Georgia",
"displayValue": "Georgia"
},
{
"value": "Hawaii",
"displayValue": "Hawaii"
},
{
"value": "Iowa",
"displayValue": "Iowa"
},
{
"value": "Idaho",
"displayValue": "Idaho"
},
{
"value": "Illinois",
"displayValue": "Illinois"
},
{
"value": "Indiana",
"displayValue": "Indiana"
},
{
"value": "Kansas",
"displayValue": "Kansas"
},
{
"value": "Kentucky",
"displayValue": "Kentucky"
},
{
"value": "Louisiana",
"displayValue": "Louisiana"
},
{
"value": "Massachusetts",
"displayValue": "Massachusetts"
},
{
"value": "Maryland",
"displayValue": "Maryland"
},
{
"value": "Maine",
"displayValue": "Maine"
},
{
"value": "Michigan",
"displayValue": "Michigan"
},
{
"value": "Minnesota",
"displayValue": "Minnesota"
},
{
"value": "Missouri",
"displayValue": "Missouri"
},
{
"value": "Mississippi",
"displayValue": "Mississippi"
},
{
"value": "Montana",
"displayValue": "Montana"
},
{
"value": "North Carolina",
"displayValue": "North Carolina"
},
{
"value": "North Dakota",
"displayValue": "North Dakota"
},
{
"value": "Nebraska",
"displayValue": "Nebraska"
},
{
"value": "New Hampshire",
"displayValue": "New Hampshire"
},
{
"value": "New Jersey",
"displayValue": "New Jersey"
},
{
"value": "New Mexico",
"displayValue": "New Mexico"
},
{
"value": "Nevada",
"displayValue": "Nevada"
},
{
"value": "New York",
"displayValue": "New York"
},
{
"value": "Ohio",
"displayValue": "Ohio"
},
{
"value": "Oklahoma",
"displayValue": "Oklahoma"
},
{
"value": "Oregon",
"displayValue": "Oregon"
},
{
"value": "Pennsylvania",
"displayValue": "Pennsylvania"
},
{
"value": "Rhode Island",
"displayValue": "Rhode Island"
},
{
"value": "South Carolina",
"displayValue": "South Carolina"
},
{
"value": "South Dakota",
"displayValue": "South Dakota"
},
{
"value": "Tennessee",
"displayValue": "Tennessee"
},
{
"value": "Texas",
"displayValue": "Texas"
},
{
"value": "Utah",
"displayValue": "Utah"
},
{
"value": "Virginia",
"displayValue": "Virginia"
},
{
"value": "Virgin Islands",
"displayValue": "Virgin Islands"
},
{
"value": "Vermont",
"displayValue": "Vermont"
},
{
"value": "Washington",
"displayValue": "Washington"
},
{
"value": "West Virginia",
"displayValue": "West Virginia"
},
{
"value": "Wisconsin",
"displayValue": "Wisconsin"
},
{
"value": "Wyoming",
"displayValue": "Wyoming"
}
]
},
"canada": {
"countryName": "canada",
"states": [
{
"value": "Alberta",
"displayValue": "Alberta"
},
{
"value": "British Columbia",
"displayValue": "British Columbia"
},
{
"value": "Manitoba",
"displayValue": "Manitoba"
},
{
"value": "New Brunswick",
"displayValue": "New Brunswick"
},
{
"value": "Newfoundland",
"displayValue": "Newfoundland"
},
{
"value": "Nova Scotia",
"displayValue": "Nova Scotia"
},
{
"value": "Northwest Territories",
"displayValue": "Northwest Territories"
},
{
"value": "Nunavut",
"displayValue": "Nunavut"
},
{
"value": "Ontario",
"displayValue": "Ontario"
},
{
"value": "Prince Edward Island",
"displayValue": "Prince Edward Island"
},
{
"value": "Quebec",
"displayValue": "Quebec"
},
{
"value": "Saskatchewan",
"displayValue": "Saskatchewan"
},
{
"value": "Yukon",
"displayValue": "Yukon"
}
]
},
};
let initialStates = [];
function captureStatesValuesFromPardot() {
$("form#pardot-form .state select option").map(function (index, item) {
initialStates.push({
"value": item.value,
"displayValue": item.innerHTML
})
return item;
})
}
function showStates() {
const initialStateValue = $('form#pardot-form .state select').val();
var t = $('form#pardot-form .country select').val();
var v = $(`form#pardot-form .country select option[value='${t}']`).html().toLowerCase().replace(" ", "");
var states = `<option value="" selected="selected"></option>`;
if (countryStateMap[v] !== null && countryStateMap[v] !== undefined) {
const selectedStatesObj = countryStateMap[v].states;
const selectedStatesArray = selectedStatesObj.map(function (item, index) {
return item.displayValue.toLowerCase().replace(" ", "");
})
const filteredStatesObj = initialStates.filter(function (item, index) {
return selectedStatesArray.includes(item.displayValue.toLowerCase().replace(" ", ""));
})
filteredStatesObj.forEach(element => {
states = states + `<option value="${element.value}">${element.displayValue}</option>`
});
$('form#pardot-form .state').show();
$('form#pardot-form .state select').html(states);
$('form#pardot-form .state select').val(initialStateValue);
$('form#pardot-form .state p.state').addClass("required");
}
else {
$('form#pardot-form .state').hide();
$('form#pardot-form .state p.state').removeClass("required");
}
}
$(document).ready(function() {
captureStatesValuesFromPardot();
showStates();
$('form#pardot-form .country select').on('change', () => {
showStates();
})
})
</script>
Step 3: Save and Test
- Save your form.
- Test the form by selecting different countries and observing how the state dropdown dynamically updates.
Conclusion
By following these steps, you can enhance your Pardot forms to display relevant state options based on the user’s country selection. Remember to customize the countryStateMap
with the appropriate country-state pairs for your specific use case. Happy form-building!